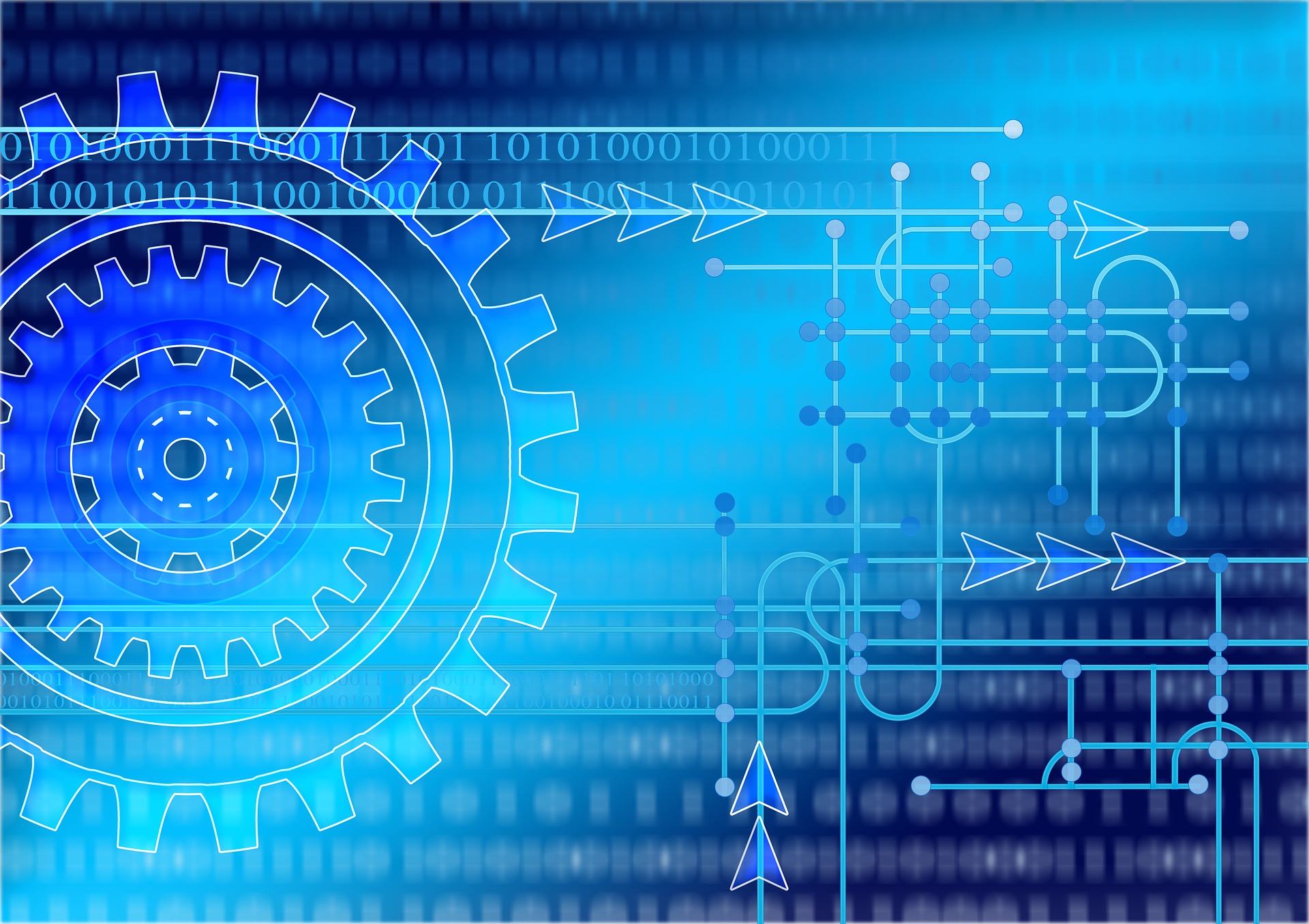
What is function at programming?
In our daily life, we do same things periodically time to time. Washing our hands and face, brushing our teeths, preparing breakfasts in the mornings, doing our job at work during all day etc. are simple examples of our routins.
At programming world, doing same tasks are the process that oftenly done. For example, you have a website that users can register. Registration is a task that will be repeated for each unique user. So, what will you do? Will you register your each new user manually by yourself?
There might be lots of tasks that need to be repeated in almost every software project. This may means more and more codes have to be written in first look, but in fact, it is not. Because there is a concept that named as “Function” in the programming literature.
As you can guess, functions are some kind of nests that contains the codes of tasks that need to be repeated when triggered.
function any_task ()
{
Write codes here...
}
This is a simple javascript function with no takes parameters and returns nothing back to caller. It just performs the task you briefed between curly braces “{ }”. As seen in the example above, they declared with the function keyword.
Functions are generally being using in the way of taking parameter, processing the task and returning the result back to the caller process. This means, you send some values into the function you’ll call, it takes the values, process the task or tasks and returns the result value(s) to you back. Let’s take a look below example.
function sum_numbers(num1, num2, num3, num4)
{
return num1+num2+num3+num4;
}
This function takes 4 parameters and returns the sum of them back to the caller event. Below code shows how to call a function with parameters.
sum_numbers(3, 7, 25, 107);
As you can see, calling a function with parameters is very easy; just write its name and declare the parameter values in the parentheses. If you won’t use parameters, just leave empty the parentheses, but DO NOT FORGET THE USE PARENTHESES ANYWAY. Even you won’t send any parameters to the function, parentheses are must be declared ALWAYS. In other saying, parentheses tell to the system that it is a “function”.
Let’s do a simple example. Create a new text document (e.g notepad) and write down the below codes. Then save the document as html by adding .html after its name.
<html>
<head>
<script>
function name_surname(n, s)
{
return n + " " + s;
}
function show_my_name(myname, mysurname)
{
alert(name_surname(myname, mysurname));
}
</script>
</head>
<body>
<div style="text-align:center; margin-top:200px;">
<p><input id="name" type="text" style="width:200px;" placeholder="Enter your name" /></p>
<p><input id="surname" type="text" style="width:200px;" placeholder="Enter your surname" />
</p>
<p><input type="button" onclick="show_my_name(document.getElementById('name').value, document.getElementById('surname').value)" value="CALL THE show_my_name FUNCTION" /></p><p>When you click this button, it will get the values of the textboxes with id "name" and "surname", then will call the function "show_my_name" with them as paramaters.</p><p>The function you called "show_my_name" will call the other function "name_surname" with the parameters you sent it and will show the returned value in a message box with the pre-built <strong>alert</strong> function.</p>
</div>
</body>
</html>



After you saved the file, you will see a document like below. Double click on it and run the file.

That’s all. You have just created two javascript functions and called them successfully. You can write much more complex and functional functions in any langauge. Although decleration keywords might be difference, all functions in all programming languages are based on the same logic.
